Outlook to PDF Conversion Automation
Universal Document Converter will convert and virtually print your documents into any of the formats of your choice: PDF, JPEG, TIFF, or PNG.
Universal Document Converter also offers numerous resources to software developers who can benefit from the software settings using COM-interface and Microsoft Outlook as COM-server to save the Outlook messages as PDF files.
Outlook message conversion source code examples:
Visual Basic.NET
VB.NET Outlook to PDF conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft Outlook version 2000 or higher should be installed and activated ' on your computer ' ' 2) Universal Document Converter 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic.NET ' ' 4) In the Visual Basic main menu press Project->Add Reference... ' ' 5) In the Add Reference window go to COM tab and double click on ' Universal Document Converter Type Library. '---------------------------------------------------------------------- Private Shared readyFlag As Boolean = False Private Shared myTimer As New System.Windows.Forms.Timer() Private Shared Sub TimerEventProcessor(ByVal myObject As Object, ByVal myEventArgs As EventArgs) ' This is the method to run when the timer is raised. myTimer.Stop() readyFlag = True End Sub Private Sub WaitSomeTime(ByVal nSec As Single) AddHandler myTimer.Tick, AddressOf TimerEventProcessor myTimer.Interval = nSec * 1000 myTimer.Start() While readyFlag = False ' Processes all the events in the queue. Application.DoEvents() End While End Sub Private Sub PrintOutlookMsgToPDF(ByVal strFilePath As String) Const olDiscard = 1 ' = Outlook.OlInspectorClose.olDiscard Dim objUDC As UDC.IUDC Dim itfPrinter As UDC.IUDCPrinter Dim itfProfile As UDC.IProfile Dim objOutlook As Object Dim itfMsg As Object objUDC = New UDC.APIWrapper itfPrinter = objUDC.Printers("Universal Document Converter") itfProfile = itfPrinter.Profile ' Set Universal Document Converter as default printer, because ' Outlook's API interface allow printing only on default printer objUDC.DefaultPrinter = "Universal Document Converter" ' Use Universal Document Converter API to change settings of converterd document itfProfile.FileFormat.ActualFormat = UDC.FormatID.FMT_PDF itfProfile.OutputLocation.Mode = UDC.LocationModeID.LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.PostProcessing.Mode = UDC.PostProcessingModeID.PP_OPEN_FOLDER ' Open MS Outlook as COM-server objOutlook = CreateObject("Outlook.Application") ' Open Outlook MSG file itfMsg = objOutlook.CreateItemFromTemplate(strFilePath) ' And print it on the default printer Call itfMsg.PrintOut() ' Close opened file itfMsg.Close(olDiscard) ' Wait until Outlook finished printing process WaitSomeTime(5) ' Close Outlook application Call objOutlook.Quit() objOutlook = Nothing End Sub
Visual Basic 6
VB Outlook to PDF conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft Outlook version 2000 or higher should be installed and activated ' on your computer ' ' 2) Universal Document Converter 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic 6.0 ' ' 4) In the Visual Basic main menu press Project->References ' ' 5) In the list of references check Universal Document Converter Type Library '---------------------------------------------------------------------- Private Sub WaitSomeTime(nSec As Single) Dim nStart, nFinish As Single nStart = Timer ' Set start time. Do While Timer < nStart + nSec DoEvents ' Yield to other processes. Loop nFinish = Timer ' Set end time. End Sub Private Sub PrintOutlookMsgToPDF(ByVal strFilePath As String) Const olDiscard = 1 ' = Outlook.OlInspectorClose.olDiscard Dim objUDC As IUDC Dim itfPrinter As IUDCPrinter Dim itfProfile As IProfile Dim objOutlook As Object Dim itfMsg As Object Set objUDC = New UDC.APIWrapper Set itfPrinter = objUDC.Printers("Universal Document Converter") Set itfProfile = itfPrinter.Profile ' Set Universal Document Converter as default printer, because ' Outlook's API interface allow printing only on default printer objUDC.DefaultPrinter = "Universal Document Converter" ' Use Universal Document Converter API to change settings of converterd document itfProfile.FileFormat.ActualFormat = FMT_PDF itfProfile.OutputLocation.Mode = LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.PostProcessing.Mode = PP_OPEN_FOLDER ' Open MS Outlook as COM-server Set objOutlook = CreateObject("Outlook.Application") ' Open Outlook MSG file Set itfMsg = objOutlook.CreateItemFromTemplate(strFilePath) ' And print it on the default printer Call itfMsg.PrintOut ' Close opened file itfMsg.Close (olDiscard) ' Wait until Outlook finished printing process WaitSomeTime (5) ' Close Outlook application Call objOutlook.Quit Set objOutlook = Nothing End Sub
Visual C++
C++ Outlook to PDF conversion code example
////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C++ starting from // Microsoft Visual Studio version 2003 or higher. // // 1. Microsoft Outlook version 2000 or higher should be installed and activated // on your computer. Please note that Microsoft Outlook Express does not have // COM interface and cannot be used as COM-server! // // 2. Universal Document Converter version 5.2 or higher should be installed as well. // // 3. You must initialize the COM before you call any COM method. // Please insert ::CoInitialize(0); in your application initialization // and ::CoUninitialize(); before closing it. // // 4. Import Office libraries for 32-bit version of Windows. // For 64-bit version please change C:\\Program Files\\ to // C:\\Program Files (x86)\\ in all pathes. #pragma message("Import MSO.DLL") // MS Office 2000 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE10\\MSO.DLL" // // MS Office 2003 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE11\\MSO.DLL" // // MS Office 2007 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE12\\MSO.DLL" #import "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE10\\MSO.DLL" \ rename_namespace("MSO"), auto_rename #pragma message("Import VBE6EXT.OLB") #import "C:\\Program Files\\Common Files\\Microsoft Shared\\VBA\\VBA6\\VBE6EXT.OLB" \ rename_namespace("VBE6EXT") #pragma message("Import MS Outlook API") // MS Office 2000 -> "C:\\Program Files\\Microsoft Office\\OFFICE\\MSOUTL9.OLB" // MS Office 2003 -> "C:\\Program Files\\Microsoft Office\\OFFICE11\\MSOUTL.OLB" // MS Office 2007 -> "C:\\Program Files\\Microsoft Office\\OFFICE12\\MSOUTL.OLB" #import "C:\\Program Files\\Microsoft Office\\OFFICE\\MSOUTL9.OLB"\ rename_namespace("OUTLOOK"), auto_rename // 5. Import Universal Document Converter software API: #import "progid:udc.apiwrapper" rename_namespace("UDC") ////////////////////////////////////////////////////////////////// #include <afx.h> void WaitSomeTime( int nSec ) { CTime tmBegin = CTime::GetCurrentTime(); do { MSG msg; while( PeekMessage( &msg, 0, 0, 0, PM_REMOVE ) ) { TranslateMessage(&msg); DispatchMessage(&msg); } } while( ( CTime::GetCurrentTime() - tmBegin ).GetTotalSeconds() < nSec ); } void PrintOutlookToPDF( CString sFilePath ) { UDC::IUDCPtr pUDC(__uuidof(UDC::APIWrapper)); UDC::IUDCPrinterPtr itfPrinter = pUDC->Printers["Universal Document Converter"]; UDC::IProfilePtr itfProfile = itfPrinter->Profile; // Set Universal Document Converter as default printer, because // Outlook's API interface allow printing only on default printer pUDC->DefaultPrinter = "Universal Document Converter"; // Use Universal Document Converter API to change settings of converterd document itfProfile->FileFormat->ActualFormat = UDC::FMT_PDF; itfProfile->OutputLocation->Mode = UDC::LM_PREDEFINED; itfProfile->OutputLocation->FolderPath = L"C:\\Out"; itfProfile->PostProcessing->Mode = UDC::PP_OPEN_FOLDER; // Run Microsoft Outlook as COM-server OUTLOOK::_ApplicationPtr objOutlook( L"Outlook.Application" ); OUTLOOK::_MailItemPtr itfMsg; // Open document from file itfMsg = objOutlook->CreateItemFromTemplate( (LPCTSTR)sFilePath ); // And print it on the default printer itfMsg->PrintOut(); // Close opened file itfMsg->Close( OUTLOOK::olDiscard ); // Wait until Outlook finished printing process WaitSomeTime( 5 ); // Close Outlook application objOutlook->Quit(); }
Visual C#
C# Outlook to PDF conversion code example:
///////////////////////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C# from // Microsoft Visual Studio version 2003 or higher. // // 1. Microsoft Outlook version 2000 or higher should be installed and activated // on your computer. Please note that Microsoft Outlook Express does not have // COM interface and cannot be used as COM-server! // // 2. Universal Document Converter version 5.2 or higher should be installed as well. // // 3. Add references to Microsoft Outlook XX.0 Object Library and // Universal Document Converter Type Library using the // Project | Add Reference menu > COM tab. // XX is the Microsoft Office version installed on your computer. ///////////////////////////////////////////////////////////////////////////////////// using System; using System.IO; using UDC; using Outlook = Microsoft.Office.Interop.Outlook; namespace OutlookToPDF { class Program { static void OutlookToPDF(string olTemplFile) { //Create a UDC object and get its interfaces IUDC objUDC = new APIWrapper(); IUDCPrinter Printer = objUDC.get_Printers("Universal Document Converter"); IProfile Profile = Printer.Profile; //Set Universal Document Converter as default printer, because //Outlook's API interface allow printing only on default printer objUDC.DefaultPrinter = "Universal Document Converter"; //Use Universal Document Converter API to change settings of //converterd document Profile.FileFormat.ActualFormat = FormatID.FMT_PDF; Profile.OutputLocation.Mode = LocationModeID.LM_PREDEFINED; Profile.OutputLocation.FolderPath = @"C:\UDC Output Files"; Profile.PostProcessing.Mode = PostProcessingModeID.PP_OPEN_FOLDER; //Run Microsoft Outlook as COM-server Outlook.Application OutlookApp = new Outlook.ApplicationClass(); //This will be passed when ever we don't want to pass value: Object Missing = Type.Missing; //Open document from file Outlook.MailItem MailItem = (Outlook.MailItem)OutlookApp.CreateItemFromTemplate(olTemplFile, Missing); //And print it on the default printer MailItem.PrintOut(); //Close opened file MailItem.Close(Outlook.OlInspectorClose.olDiscard); //Wait until Outlook finished printing process System.Threading.Thread.Sleep(5000); //Close Outlook application OutlookApp.Quit(); } static void Main(string[] args) { string TemplateFilePath = @"c:\Docs\Test.msg"; OutlookToPDF(TemplateFilePath); } } }
Delphi
Delphi Outlook to PDF conversion code example:
/////////////////////////////////////////////////////////////////////////////////// // This example was designed to be used in Delphi 7 or higher. // // 1. Microsoft Outlook version 2000 or higher should be installed and activated // on your computer. Please note that Microsoft Outlook Express does not have // COM interface and cannot be used as COM-server! // // 2. Universal Document Converter version 5.2 or higher should be installed as well. // // 3. Add Universal Document Converter Type Library and // Microsoft Outlook XX.0 Object Library type libraries to the project. // XX is the Microsoft Office version installed on your computer. // // Delphi 7: // Use the Project | Import Type Library menu. // // Delphi 2006 or latter: // Use the Component | Import Component menu. // // Clear the Generate Component Wrapper checkbox and click the Create Unit // button (Delphi 7) or select the Create Unit option (Delphi 2006 or latter). // // 4. Notice that the number of Microsoft Outlook's method parameters may depend // on the Outlook version. /////////////////////////////////////////////////////////////////////////////////// program OutlookToPDF; {$APPTYPE CONSOLE} uses SysUtils, Variants, Dialogs, ActiveX, Windows, UDC_TLB, Outlook_TLB; procedure PrintOutlookToPDF(OutlookFilePath: string); var objUDC: IUDC; Printer: IUDCPrinter; Profile: IProfile; OutlookApp: OutlookApplication; MailItem: Outlook_TLB.MailItem; ReadOnly: OleVariant; Missing: OleVariant; begin //Create a UDC object and get its interfaces objUDC := CoAPIWrapper.Create; Printer := objUDC.get_Printers('Universal Document Converter'); Profile := Printer.Profile; //Use Universal Document Converter API to change settings of convertered document Profile.PageSetup.ResolutionX := 300; Profile.PageSetup.ResolutionY := 300; //Set Universal Document Converter as default printer, because //Outlook's API interface allow printing only on default printer objUDC.DefaultPrinter := 'Universal Document Converter'; //Use Universal Document Converter API to change settings of converterd document Profile.FileFormat.ActualFormat := FMT_PDF; Profile.OutputLocation.Mode := LM_PREDEFINED; Profile.OutputLocation.FolderPath := 'C:\UDC Output Files'; Profile.PostProcessing.Mode := PP_OPEN_FOLDER; //Run Microsoft Outlook as COM-server OutlookApp := CoOutlookApplication.Create; ReadOnly := True; //This will be passed when ever we don't want to pass value: Missing := Variants.EmptyParam; //Open document from file MailItem := OutlookApp.CreateItemFromTemplate(OutlookFilePath, Missing) as Outlook_TLB.MailItem; //And print it on the default printer MailItem.PrintOut(); //Close opened file MailItem.Close(olDiscard); //Wait until Outlook finished printing process Sleep(5000); //Close Outlook application OutlookApp.Quit(); end; var TestFilePath: string; begin TestFilePath := ExtractFilePath(ParamStr(0)) + 'TestFile.msg'; CoInitialize(nil); try PrintOutlookToPDF(TestFilePath); finally CoUninitialize; end; end.
PHP
PHP Outlook to PDF converter code example:
'---------------------------------------------------------------------- ' 1) Microsoft Outlook 2000 or above should be installed and activated ' on your computer. ' ' 2) Universal Document Converter 5.2 or above should also be installed. ' ' 3) Apache WEB server and PHP 4.0 or above should be installed and adjusted. '---------------------------------------------------------------------- <?PHP //Create Universal Document Converter object $objUDC = new COM("UDC.APIWrapper"); //Set up Universal Document Converter $itfPrinter = $objUDC->Printers("Universal Document Converter"); $itfProfile = $itfPrinter->Profile; $itfProfile->PageSetup->ResolutionX = 300; $itfProfile->PageSetup->ResolutionY = 300; $itfProfile->PageSetup->Orientation = 0; $itfProfile->PageSetup->Units = 1; $itfProfile->PageSetup->Width = 220; $itfProfile->PageSetup->Height = 180; $itfProfile->FileFormat->ActualFormat = 7; //PDF $itfProfile->OutputLocation->Mode = 1; $itfProfile->OutputLocation->FolderPath = '&[Documents]\UDC Output Files\\'; $itfProfile->OutputLocation->FileName = '&[DocName(0)].&[ImageType]'; $itfProfile->OutputLocation->OverwriteExistingFile = 1; $itfProfile->Adjustments->Crop->Mode = 0; $itfProfile->PostProcessing->Mode = 0; $itProfile->ShowProgressWnd = 1; //Create MS Outlook object and export the file $file = 'my_mail.msg'; $Outlook = new COM("Outlook.Application"); $Msg = $Outlook->CreateItemFromTemplate($file); //Set UDC as default printer, and save the previous default printer as variable $DefPrinter = $objUDC->DefaultPrinter; $objUDC->DefaultPrinter = "Universal Document Converter"; //Printing $Msg->PrintOut(); //Pause for processing sleep(5); //Close the document $Msg->Close(0); //Close Outlook $Outlook->Quit; //Set previous printer as default $objUDC->DefaultPrinter = $DefPrinter; echo "READY!"; ?>
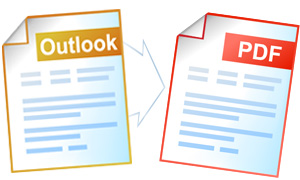